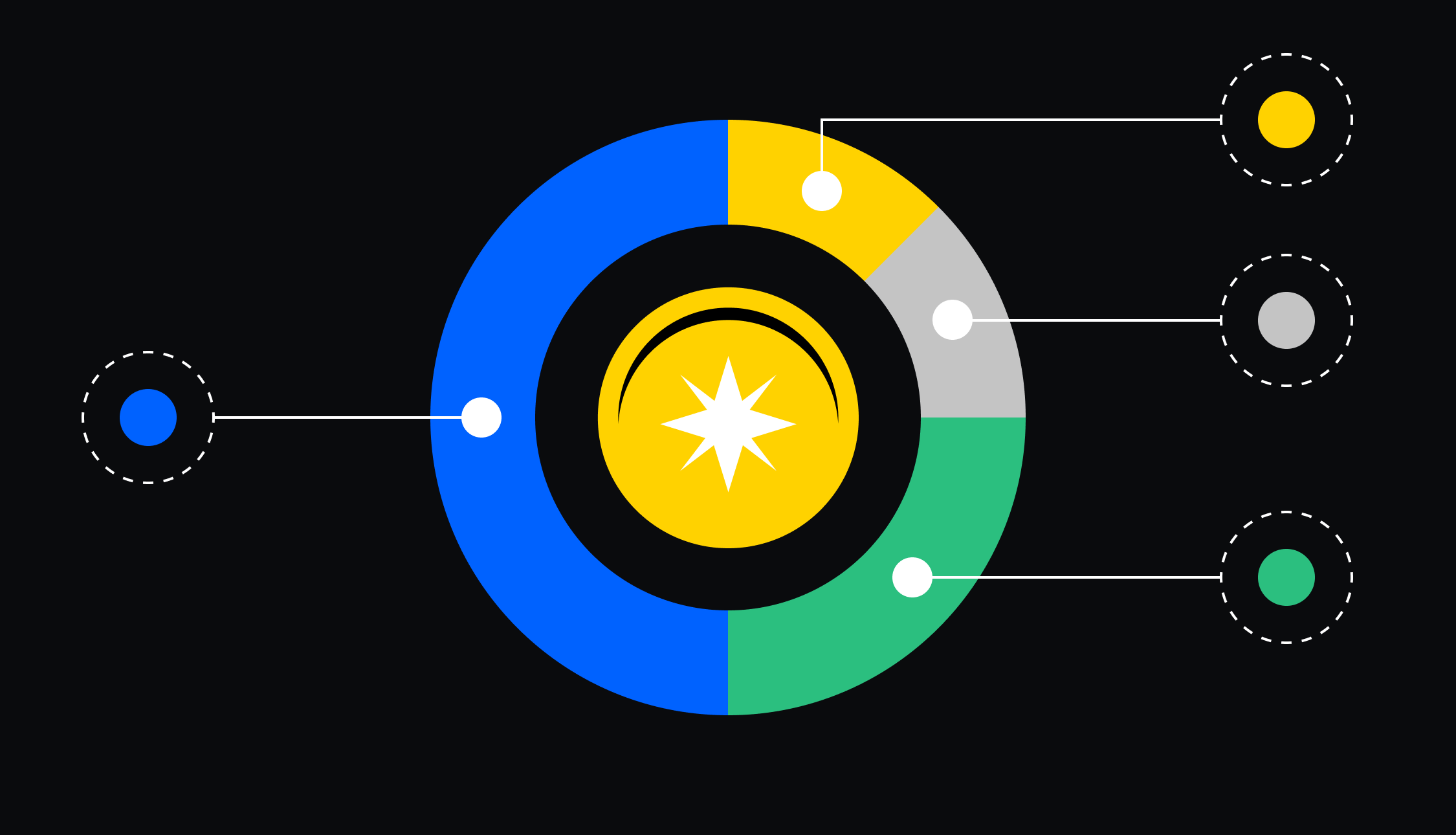
Crypto trading moves fast—sometimes too fast for human hands. That’s where trading bots come in, automating trades with precision and speed. If you’re ready to take control of your crypto game, building your own trading bot is the way to go. This isn’t just about slapping some code together; it’s about mastering algorithms and architecture to create a tool that works for you. In this post, we’re breaking down the nuts and bolts of crypto trading bot development—how to design it, what makes it tick, and why it matters in today’s market. Let’s get started!
Why Build a Crypto Trading Bot?
First off, why bother? The crypto market never sleeps. Bitcoin hit $69,000 in November 2021, then crashed to $16,000 a year later, according to CoinMarketCap data. Prices swing hard, and opportunities vanish in seconds. A trading bot doesn’t blink—it executes trades based on logic, not emotion. Studies from 2023 show automated trading systems can increase profitability by up to 20% compared to manual trading, per research from the Journal of Financial Markets. That’s real money on the table.
Plus, it’s not just about trading crypto. If you’re into Crypto Token Development, a bot can help you monitor token performance or execute strategies for your project. Partnering with a Token Development Company might get you the token, but a custom bot keeps it moving in the market. Let’s explore how to make this happen.
The Core of Crypto Trading Bot Development
At its heart, a trading bot is a program that connects to an exchange, analyzes data, and trades based on rules you set. Sounds simple, right? It’s not. The magic lies in two pillars: algorithms and architecture. Get these right, and you’ve got a bot that can scalp profits or ride trends. Mess them up, and you’re bleeding funds faster than a bad margin call.
Algorithms: The Brain of Your Bot
Algorithms decide when to buy, sell, or hold. They’re the logic driving your bot, and they need to be sharp. Here are some key types to consider:
1. Moving Average Crossovers
This is a classic. You take two moving averages—say, a 50-day and a 200-day. When the short-term crosses above the long-term, it’s a buy signal. Below? Sell. A 2022 study by CryptoCompare found that simple moving average strategies delivered a 15% annualized return on Bitcoin during volatile periods. It’s basic but effective.
2. Arbitrage
Crypto exchanges don’t always agree on price. On March 1, 2025, Binance might list ETH at $2,500 while Kraken has it at $2,510. An arbitrage bot spots that gap, buys low, sells high, and pockets the difference. Speed matters here—latency can kill profits. Data from Chainalysis shows arbitrage accounted for 5% of crypto trading volume in 2024.
3. Machine Learning Models
Want to level up? Use machine learning (ML). Feed your bot historical price data, and it learns patterns. A 2023 MIT study showed ML-based trading bots outperformed static strategies by 12% in bear markets. Libraries like TensorFlow or PyTorch make this doable, but it’s not plug-and-play—you’ll need clean data and patience.
Pick an algorithm that fits your goals. Scalping? Go arbitrage. Long-term gains? Moving averages or ML. If you’re testing tokens from a Crypto Token Development project, tweak the algorithm to prioritize volume or price stability.
Architecture: The Skeleton of Your Bot
Algorithms are useless without a solid structure. Your bot’s architecture is how it’s built—how it connects, processes, and acts. Here’s the breakdown:
1. Data Feed Module
Your bot needs real-time data—prices, volumes, order books. Most exchanges (Binance, Coinbase, Kraken) offer APIs for this. For example, Binance’s WebSocket API pushes updates in milliseconds. A 2024 report from Kaiko found that 80% of professional traders rely on API-driven data. If you’re slow here, you’re toast.
2. Strategy Engine
This is where your algorithm lives. It takes data, crunches numbers, and spits out decisions. Keep it modular—separate the logic from the execution—so you can swap strategies without rebuilding everything.
3. Order Execution Module
Once the strategy says “buy,” this part talks to the exchange and makes it happen. Timing is critical. A 2023 study by the Blockchain Research Lab found that execution delays over 500ms cut profitability by 8%. Use asynchronous programming (like Python’s asyncio) to keep it snappy.
4. Risk Management Layer
Bots don’t care about your wallet—unless you tell them to. Add stop-loss limits or position sizing rules. During the May 2021 crash, traders with automated risk controls lost 30% less than those without, per Glassnode data.
5. Logging & Monitoring
You need to know what’s happening. Log every trade, error, and decision. Tools like Prometheus or Grafana can track performance live. If your bot’s tied to a Token Development Company project, this data proves its worth to stakeholders.
Tools You’ll Need
Ready to build? You don’t need a PhD, but you do need the right gear. Here’s what works:
- Programming Language: Python’s king—easy to learn, tons of libraries (Pandas for data, ccxt for exchange APIs). A 2024 Stack Overflow survey showed 60% of crypto devs use it.
- Exchange APIs: Binance, Coinbase, KuCoin—pick one with good docs and low fees. Binance handles 1.4 million transactions per second, per their 2024 stats.
- Cloud Hosting: AWS or Google Cloud keep your bot running 24/7. A VPS costs $5-$20/month—cheap insurance against power outages.
- Testing Framework: Backtest with historical data first. Platforms like Backtrader or QuantConnect let you simulate trades without risking cash.
If you’re working on Crypto Token Development, integrate your bot with a blockchain explorer API (like Etherscan) to track token-specific metrics.
Step-by-Step: Building Your Bot
Let’s walk through it. This isn’t theory—it’s a roadmap you can follow today.
Step 1: Define Your Strategy
Say you choose a moving average crossover. You’ll need 50-day and 200-day averages. Code it to buy when the 50-day crosses above, sell when it dips below. Test it on BTC/USD data from 2024—CoinGecko offers free historical sets.
Step 2: Set Up the Data Feed
Grab an API key from Binance. Use Python’s ccxt library:
import ccxt
exchange = ccxt.binance({'apiKey': 'your_key', 'secret': 'your_secret'})
ticker = exchange.fetch_ticker('BTC/USDT')
print(ticker['last'])
This pulls the latest BTC price. Hook it to a WebSocket for real-time updates.
Step 3: Code the Strategy Engine
Calculate those averages:
import pandas as pd
data = pd.DataFrame(exchange.fetch_ohlcv('BTC/USDT', '1d'))
data['MA50'] = data['close'].rolling(50).mean()
data['MA200'] = data['close'].rolling(200).mean()
If MA50 > MA200, buy. Reverse? Sell.
Step 4: Execute Trades
Use the API to place orders:
exchange.create_market_buy_order('BTC/USDT', 0.001) # Buy 0.001 BTC
Add error handling—APIs fail sometimes.
Step 5: Backtest & Deploy
Run it against 2024 data. Did it profit? Tweak it. Then deploy on a cloud server. Monitor it daily—markets shift, and bots need updates.
Real-World Challenges
It’s not all smooth sailing. Exchanges charge fees—Binance takes 0.1% per trade, eating into profits. Latency can lag you behind high-frequency traders. And bugs? One typo in 2023 cost a dev $50,000 when his bot sold at a loss, per a Reddit autopsy. Test hard before going live.
If you’re tying this to Crypto Token Development, liquidity’s another hurdle. New tokens often lack volume, so your bot might sit idle. A Token Development Company can advise on market-making, but your bot still needs smart rules to avoid dead zones.
Scaling Up: Beyond the Basics
Mastered the simple bot? Add complexity. Integrate sentiment analysis from X posts—crypto Twitter moves markets. A 2024 study by SentimentTrader linked 10% price jumps to viral tweets. Or plug in ML to predict volatility—studies show it’s 70% accurate on 7-day forecasts, per IEEE research.
For token projects, build a bot that interacts with smart contracts. A Token Development Company might handle the token, but your bot could automate staking or liquidity provision—real utility that boosts value.
The Payoff
Here’s the kicker: a well-built bot can run 24/7, catching opportunities you’d miss. In 2024, automated traders averaged 18% returns versus 11% for manual traders, per CryptoQuant. That’s not luck—that’s engineering. Whether you’re trading BTC or launching a token with Crypto Token Development, this skill pays off.
Final Thoughts
Building a crypto trading bot isn’t a weekend project—it’s a craft. Nail the algorithms, structure the architecture, and you’ve got a machine that works while you sleep. Start small, test rigorously, and scale smart. The market’s a beast, but with the right bot, you can tame it. Got questions? Drop them below—let’s keep this rolling!
Leave a Reply